1. Android中常用布局整理
1.1 LinearLayout(线性布局)
- orientation:布局方向(horizontal 水平或 vertical 垂直)。
- gravity:控制子元素在布局中的位置,如 center、left、right、top、bottom、center_vertical、center_horizontal 等。
- layout_gravity:控制组件相对于父布局的位置,比如 center、center_vertical、center_horizontal 等。
- weight:在布局中分配剩余空间的比例。
- margin:组件外部的间距,可以有 marginLeft、marginRight、marginTop、marginBottom。
- padding:组件内部的间距,包含内容与组件边界的间距。
1.2 RelativeLayout(相对布局)
- layout_above:放置在指定元素之上。
- layout_below:放置在指定元素之下。
- layout_toLeftOf:放置在指定元素的左边。
- layout_toRightOf:放置在指定元素的右边。
- layout_alignParentTop:与父元素顶部对齐。
- layout_alignParentBottom:与父元素底部对齐。
- layout_alignParentLeft:与父元素左边对齐。
- layout_alignParentRight:与父元素右边对齐。
- layout_centerHorizontal:水平居中。
- layout_centerVertical:垂直居中。
- layout_centerInParent:在父元素中心居中。
- layout_alignBaseline:与指定元素的基线对齐。
- layout_alignStart:与指定元素的开始位置对齐。
- layout_alignEnd:与指定元素的结束位置对齐。
1.3 FrameLayout(帧布局)
- layout_gravity:控制子元素的位置,与 LinearLayout 中的 gravity 类似。
- layout_marginTop、layout_marginBottom、layout_marginLeft、layout_marginRight:设置子视图的上、下、左、右外边距。
- padding:设置子视图内部与边界的间隔。
帧布局是一种简单的布局,用于堆叠视图。默认情况下,所有的子视图都会被定位在布局的左上角,但可以通过使用 layout_gravity 属性来改变它们的位置。
2.Context
2.1 简介
Context
在Android应用开发中非常关键,它提供了一个窗口,让应用程序可以访问应用程序以外的资源、服务和系统级操作。
- 资源访问:
- 访问资源文件:使用
Context
可以访问 res
目录下的各种资源,如字符串、颜色和尺寸等。
- 获取视图:在Activity中,你可以通过
findViewById
方法来获取布局文件中定义的视图。
- 权限检查:
- 运行时权限:从 Android 6.0 开始,可以调用
Context
的 checkSelfPermission
方法来检查权限。
- 系统服务:
- 获取系统服务:可以通过
Context
获取系统级服务,如 LocationManager
、WifiManager
等。
- ……
2.2 基础运用
2.2.1 通过context对象获取私有目录
私有目录也叫沙箱目录
1
| context.getFilesDir().getPath()
|
1 2 3 4 5 6 7 8 9
| public class MainActivity extends AppCompatActivity {
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); System.out.println(this.getFilesDir().getPath()); } }
|
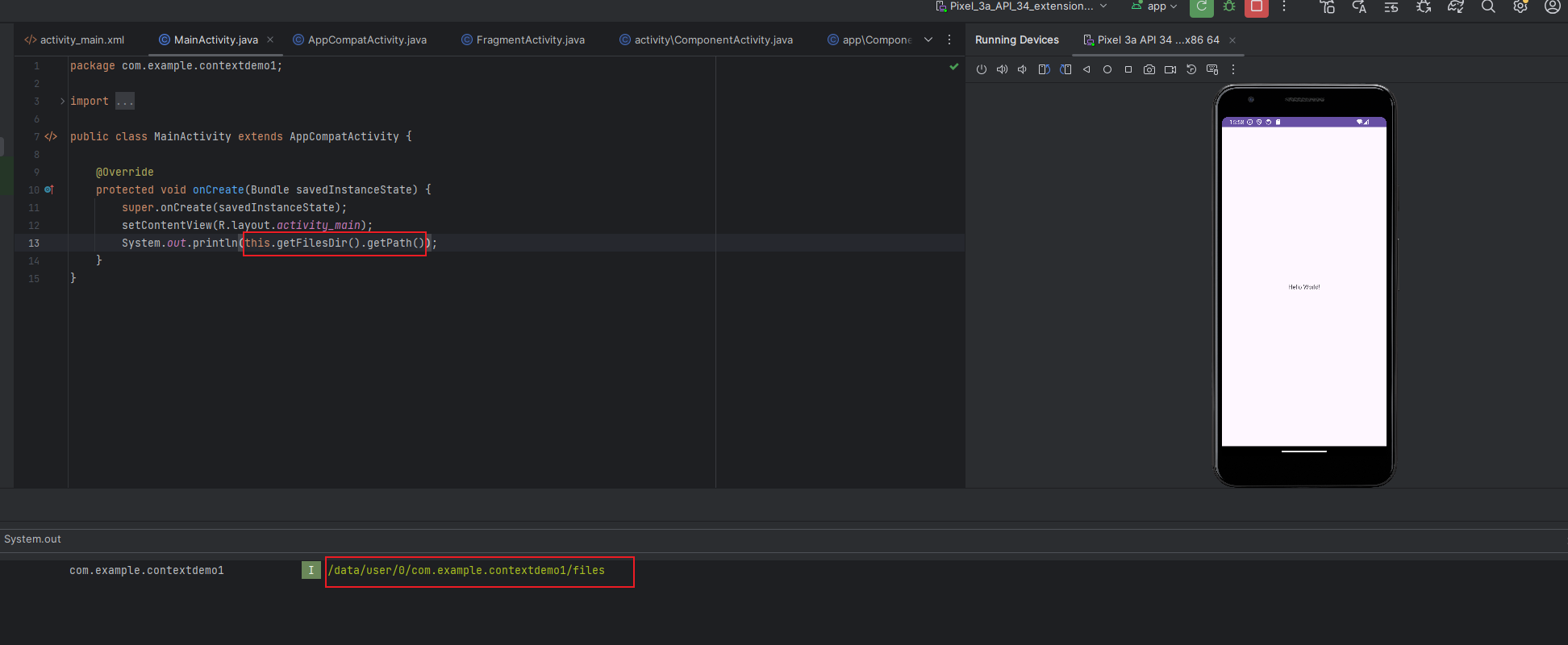
使用this.getFilesDir().getPath(),是因为this指代当前的MainActivity对象,MainActivity本质是继承context的,所以可以通过this调用context相关的api
实际开发中,为了方便context对象的传递,建议使用以下写法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| package com.example.contextdemo1;
import androidx.appcompat.app.AppCompatActivity; import android.content.Context; import android.os.Bundle;
public class MainActivity extends AppCompatActivity { private Context mContext; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mContext = this; System.out.println(mContext.getFilesDir().getPath()); } }
|
3. 练习案例-登录并保存账密
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
| package com.example.logindemo1;
import androidx.appcompat.app.AppCompatActivity; import android.content.Context; import android.os.Bundle; import android.text.TextUtils; import android.view.View; import android.widget.Button; import android.widget.CheckBox; import android.widget.EditText; import android.widget.Toast; import java.io.IOException;
public class MainActivity extends AppCompatActivity {
private EditText et_username; private EditText et_password; private CheckBox cb_rem;
private Context mContext;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mContext = this; et_username = findViewById(R.id.et_username); et_password = findViewById(R.id.et_password); cb_rem = findViewById(R.id.cb_rem); Button bt_login = findViewById(R.id.bt_login);
bt_login.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { String username = et_username.getText().toString().trim(); String password = et_password.getText().toString().trim(); try { login(mContext,username,password); } catch (IOException e) { throw new RuntimeException(e); } } }); }
private void login(Context mContext, String username, String password) throws IOException { if(!(TextUtils.isEmpty(username) || TextUtils.isEmpty(password))){ Boolean loginResult = checkLogin(mContext,username,password); System.out.println(cb_rem.isChecked()); if(cb_rem.isChecked() && loginResult.equals(true)){ mContext.openFileOutput("username.txt", Context.MODE_PRIVATE).write(username.getBytes()); mContext.openFileOutput("password.txt", Context.MODE_PRIVATE).write(password.getBytes()); } }else { Toast.makeText(mContext,"账号或密码不能为空",Toast.LENGTH_LONG).show(); } }
private Boolean checkLogin(Context mContext, String username, String password) { if(username.equals("admin")&&password.equals("123456")){ Toast.makeText(mContext,"登录成功",Toast.LENGTH_LONG).show(); return true; }else{ Toast.makeText(mContext,"账号或密码错误",Toast.LENGTH_LONG).show(); return false; } } }
|